Introduction
Redux middleware is a powerful feature that allows you to extend the capabilities of Redux. Middleware sits between the dispatching of an action and the moment it reaches the reducer, enabling you to intercept and act upon actions, perform asynchronous operations, and implement various cross-cutting concerns such as logging, crash reporting, and more.
In this article, we will explore the concept of Redux middleware, understand how it works, and learn how to create and apply custom middleware in a Redux application.
What is Redux Middleware?
Redux middleware provides a third-party extension point between dispatching an action and the moment it reaches the reducer. Middleware can:
- Intercept actions before they reach the reducer.
- Perform side effects such as data fetching or logging.
- Dispatch other actions based on the intercepted actions.
How Middleware Works
Middleware is essentially a function that takes the store
object, a next
function, and an action
, and returns a function. Here’s a basic example:
const exampleMiddleware = store => next => action => {
console.log('Dispatching:', action);
let result = next(action);
console.log('Next State:', store.getState());
return result;
};
In this example:
store
gives access to the Redux store.next
is a function that passes the action to the next middleware in the chain.action
is the action being dispatched.
Applying Middleware
To apply middleware, you use the applyMiddleware
function from Redux. However, with Redux Toolkit, middleware setup is simplified using configureStore
.
Example: Applying a Logger Middleware
First, let’s create a logger middleware:
const loggerMiddleware = store => next => action => {
console.log('Dispatching:', action);
let result = next(action);
console.log('Next State:', store.getState());
return result;
};
Next, integrate this middleware into the Redux store configuration:
import { configureStore } from '@reduxjs/toolkit';
import rootReducer from './rootReducer'; // Assuming you have a rootReducer
export const store = configureStore({
reducer: rootReducer,
middleware: (getDefaultMiddleware) => getDefaultMiddleware().concat(loggerMiddleware),
});
export default store;
Creating Custom Middleware
Custom middleware can be tailored to specific needs such as error handling, authentication, or any side effect management.
Example: Error Handling Middleware
Let’s create an error handling middleware that logs errors and dispatches an action to show a notification:
const errorHandlingMiddleware = store => next => action => {
if (action.type.endsWith('rejected')) {
console.error('Error:', action.error);
store.dispatch({ type: 'notifications/show', payload: action.error.message });
}
return next(action);
};
Integrate this middleware similarly:
import { configureStore } from '@reduxjs/toolkit';
import rootReducer from './rootReducer';
import loggerMiddleware from './loggerMiddleware';
import errorHandlingMiddleware from './errorHandlingMiddleware';
export const store = configureStore({
reducer: rootReducer,
middleware: (getDefaultMiddleware) => getDefaultMiddleware().concat(loggerMiddleware, errorHandlingMiddleware),
});
export default store;
Thunk Middleware for Asynchronous Actions
One of the most common uses of middleware is handling asynchronous actions. Redux Thunk middleware allows you to write action creators that return a function instead of an action. This function can perform side effects and dispatch actions based on the outcome.
Example: Using Thunk Middleware
Thunk middleware is included by default with Redux Toolkit. Here’s how you can use it to fetch data from an API:
import { createSlice, createAsyncThunk } from '@reduxjs/toolkit';
import axios from 'axios';
// Async thunk to fetch data
export const fetchData = createAsyncThunk('data/fetchData', async () => {
const response = await axios.get('https://api.example.com/data');
return response.data;
});
const dataSlice = createSlice({
name: 'data',
initialState: { items: [], status: 'idle', error: null },
reducers: {},
extraReducers: (builder) => {
builder
.addCase(fetchData.pending, (state) => {
state.status = 'loading';
})
.addCase(fetchData.fulfilled, (state, action) => {
state.status = 'succeeded';
state.items = action.payload;
})
.addCase(fetchData.rejected, (state, action) => {
state.status = 'failed';
state.error = action.error.message;
});
},
});
export default dataSlice.reducer;
Advanced Middleware: Combining Multiple Middleware
In real-world applications, you often need to combine multiple middleware to handle different concerns. Redux Toolkit allows you to easily concatenate multiple middleware.
Example: Combining Logger, Error Handling, and Thunk Middleware
Here’s how you can combine different middleware:
import { configureStore } from '@reduxjs/toolkit';
import rootReducer from './rootReducer';
import loggerMiddleware from './loggerMiddleware';
import errorHandlingMiddleware from './errorHandlingMiddleware';
export const store = configureStore({
reducer: rootReducer,
middleware: (getDefaultMiddleware) => getDefaultMiddleware().concat(
loggerMiddleware,
errorHandlingMiddleware,
),
});
export default store;
Middleware Best Practices
- Keep Middleware Pure: Middleware should ideally be pure functions, meaning they should not have side effects other than those they are explicitly designed to handle.
- Error Handling: Implement comprehensive error handling within your middleware to ensure that unexpected issues do not crash your application.
- Testing: Write tests for your middleware to ensure they behave as expected. This can include unit tests for individual middleware and integration tests for middleware chains.
- Performance: Be mindful of the performance implications of your middleware, especially if it involves intensive computations or frequent logging.
Conclusion
Redux middleware is a versatile and powerful tool that extends the functionality of your Redux store. By understanding and leveraging middleware, you can handle side effects, perform asynchronous operations, and implement sophisticated state management patterns in your applications. With Redux Toolkit, integrating middleware into your Redux setup is straightforward, allowing you to focus on building robust and maintainable applications.
Resources:
Happy coding!
真诚赞赏,手留余香
使用微信扫描二维码完成支付
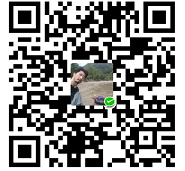